This is a follow up post to the introduction to ROS and the Beagle Bone Blue (BBBL). I will guide you through compiling your first own ROS node which contains some functionality of the robotcontrol library. This library is specifically made for the BBBL and enables the robotics functionality of the board (I/O ports, I2C, PWM, UART, etc.).
It usually comes with the preinstalled images if you followed the previous post. Otherwise, you can install it with
$ sudo apt-get install librobotcontrol
Writing a simple C program
First things first, we will write a super simple C-Program, to see if everything is set up correctly. Open up your favorite text-editor on the BBBL (mine is Vim of course) and paste/write the following simple hello world. Besides the text appearing on stdout, it will also light up the green on-board LED and then turn it off again after some time.
#include <stdio.h>
#include <rc/led.h>
#include <rc/time.h>
#define WAIT_US 500000 // time to light each LED in microseconds
int main()
{
printf("Hello, World!\n");
rc_led_set(RC_LED_GREEN,1);
rc_usleep(WAIT_US);
rc_led_set(RC_LED_GREEN,0);
rc_led_cleanup();
return 0;
}
Compile the program with
$ gcc test.c -lrobotcontrol
and run the output. Congratulations if you see the LED lighten up!
Writing your first ROS C++ program
All the source code is for this section is available on my github. We will roughly follow the talker / listener tutorial described in the official ROS tutorials but add our own code to use the robotics capabilities of the BBBL.
To do this, modify the talker.cpp file as shown below, which adds a line for turning the green LED on and off and includes the necessary header file.
#include "ros/ros.h"
#include "std_msgs/String.h"
#include <sstream>
#include <rc/led.h>
int main(int argc, char **argv)
{
ros::init(argc, argv, "talker");
ros::NodeHandle n;
ros::Publisher chatter_pub = n.advertise<std_msgs::String>("chatter", 1000);
ros::Rate loop_rate(100);
int count = 0;
while (ros::ok())
{
rc_led_set(RC_LED_GREEN, count % 2);
std_msgs::String msg;
std::stringstream ss;
ss << "hello world " << count;
msg.data = ss.str();
ROS_INFO("%s", msg.data.c_str());
chatter_pub.publish(msg);
ros::spinOnce();
loop_rate.sleep();
++count;
}
rc_led_set(RC_LED_GREEN, 0);
return 0;
}
For building the project, we have to add the line
target_link_libraries(talker robotcontrol)
to the CMakeLists.txt file of the package. Then build the package as always utilizing the catkin_make
command. Next, use rosrun to start the talker and listener after starting a roscore on the BBBL and you should get the usual talker listener helloworld output as well as a blinking green LED. Cool!
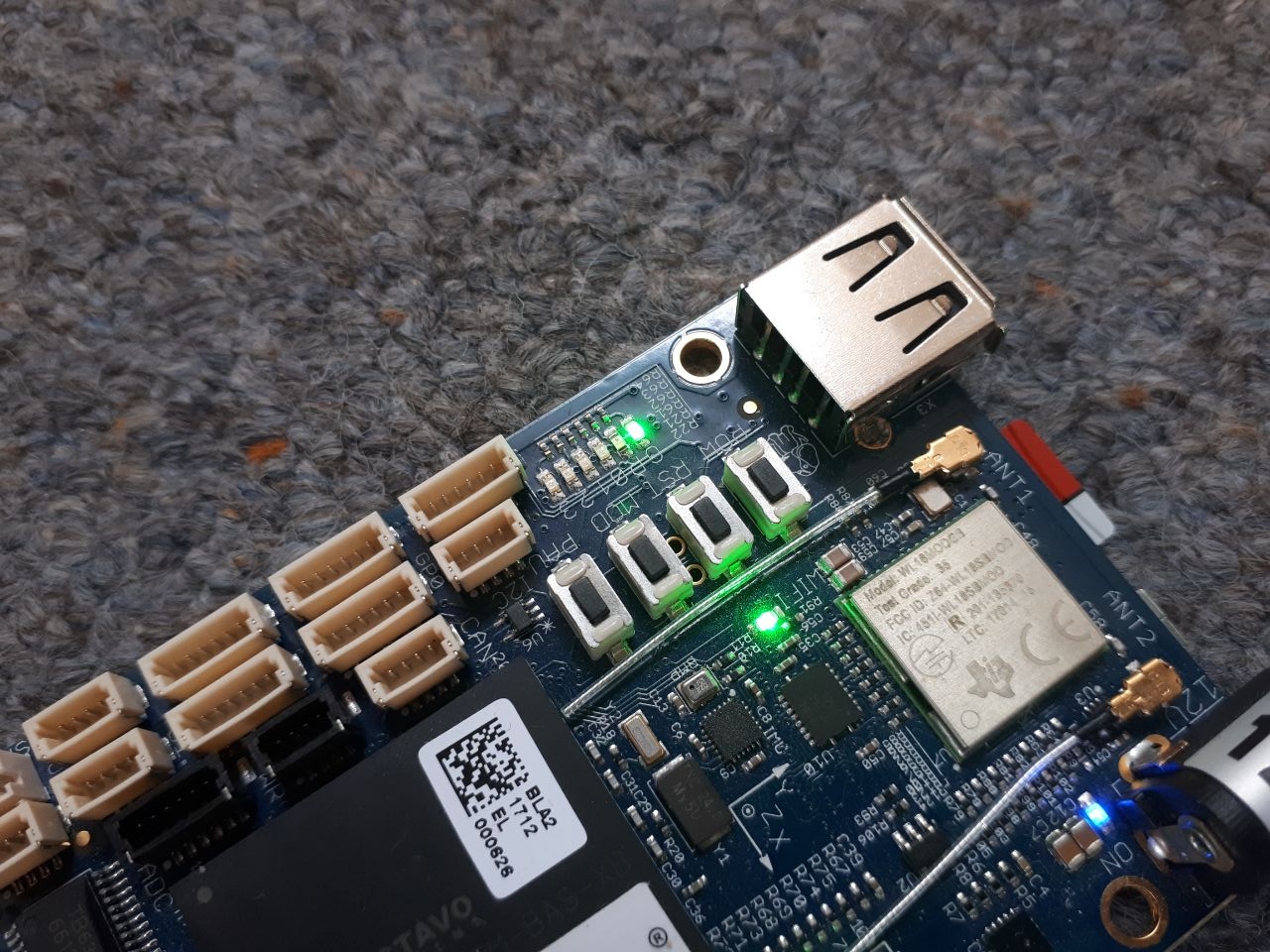